How to Read in Every Nth Line in Matlab

MATLAB Functions -- Basic Features
- Functions versus Scripts
- Anatomy of a MATLAB office
- Creating part m-files with a plain text editor
- Function Defintion
- Input and Output parameters
- Comment statements
- Some simple examples
- Calling MATLAB Functions
- Local Variables
- Flow Control
- if constructs
- Logical comparisons
- while constructs
- for constructs
Functions versus Scripts
Run across Scripts versus Functions.Anatomy of a MATLAB office
MATLAB functions are similar to C functions or Fortran subroutines.MATLAB programs are stored equally apparently text in files having names that terminate with the extension ``.k''. These files are called, not surprisingly, m-files. Each m-file contains exactly one MATLAB function. Thus, a collection of MATLAB functions can lead to a large number of relatively small files.
One nifty divergence between MATLAB and traditional high level languages is that MATLAB functions can be used interactively. In addition to providing the obvious back up for interactive calculation, it also is a very convenient way to debug functions that are part of a bigger project.
MATLAB functions have two parameter lists, i for input and i for output. This supports one of the cardinal rules of MATLAB programming: don't modify the input parameters of a role. Like all fundamental rules, this 1 is broken at times. My free communication, however, is to stick to the rule. This will require you to make some slight adjustments in the style y'all program. In the terminate this shift will assist y'all write ameliorate MATLAB code.
Creating part yard-files with a evidently text editor
MATLAB m-files must exist plain text files, i.e. files with none of the special formatting characters included by default in files created past word-processors. Nigh word-processors provide the option of saving the file as evidently text, (wait for a ``Save As...'' option in the file menu). A word-processor is overkill for creating 1000-files, nonetheless, and it is usually more user-friendly to use a simple text editor, or a ``programmer'due south editor''. For almost types of computers at that place are several text editors (often as freeware or shareware). Commonly one plain text editor is included with the operating system.When you are writing m-files yous will usually want to have the text editor and MATLAB open at the same time. Since modern word-processors require lots of system RAM it may not fifty-fifty exist possible or practical (if yous are working on a stand up-alone personal computer) for you to utilize a word-processor for g-file development. In this case a uncomplicated, text editor will be your merely selection.
Role Defintion
The first line of a role m-file must be of the following form.function [output_parameter_list] = function_name(input_parameter_list)The starting time word must ever be ``function''. Post-obit that, the (optional) output parameters are enclosed in square brackets [ ]. If the function has no output_parameter_list the square brackets and the equal sign are as well omitted. The function_name is a graphic symbol string that volition be used to call the role. The function_name must also be the same as the file name (without the ``.m'') in which the role is stored. In other words the MATLAB function, ``foo'', must be stored in the file, ``foo.grand''. Following the file name is the (optional) input_parameter_list.
At that place can exactly be i MATLAB role per yard-file.
Input and Output parameters
The input_parameter_list and output_parameter_list are comma-separated lists of MATLAB variables.Unlike other languages, the variables in the input_parameter_list should never exist altered by the statements within the role. Expert MATLAB programmers take means and reasons for violating that principle, but it is good practice to consider the input variables to be constants that cannot be inverse. The separation of input and output variables helps to reinforce this principle.
The input and output variables tin can be scalars, vectors, matrices, and strings. In fact, MATLAB does not really distinguish between variables types until some calculation or operation involving the variables is performed. It is perfectly acceptable that the input to a function is a scalar during one call and a vector during some other call.
To make the preceding betoken more concrete, consider the following statement
>> y = sin(ten)which is a call to the built-in sine office. If x is a scalar (i.e. a matrix with one row and one column) then y will be a scalar. If x is a row vector, and so y volition exist a row vector. If x is a matrix then y is a matrix. (Y'all should verify these statements with some elementary MATLAB calculations.)
This situation-dependence of input and output variables is a very powerful and potentially very confusing feature of MATLAB. Refer to the ``addtwo.g'' function below for an example.
Comment statements
MATLAB comment statements begin with the per centum graphic symbol, %. All characters from the % to the finish of the line are treated as a annotate. The % grapheme does not demand to exist in column 1.Some uncomplicated examples
Here is a piffling function, addtwo.mpart addtwo(10,y) % addtwo(10,y) Adds two numbers, vectors, whatever, and % print the result = x + y 10+yThe
addtwo
function has no output parameters and so the square brackets and the equal sign are omitted. There is only one MATLAB statement, 10+y
, in the function. Since this line does not terminate with a semicolon the results of the calculation are printed to the control window. The start ii lines after the function definition are comment statements. Not only practice these statements depict the statements in the file, their position in the file supports the on-line help facility in MATLAB. If the first line of a MATLAB function definition is immediately followed by non-blank comment statements, then those annotate statements are printed to the control window when you lot type ``assistance function_name". Endeavour it with the addtwo role. MATLAB volition impress up until a bare line or an executable statement, whichever comes start. Refer to the Role prologues -- providing assist section for more than data.
To test your agreement of input and output variables, laissez passer the post-obit definitions of 10 and y to the addtwo
function. (To save space the x and y variables are defined on the aforementioned line. Yous tin can enter these variables on the aforementioned line, as shown, or use separate lines.)
>> 10 = 2; y = 3; >> x = [2 three]; y = [four; 5]; >> x = center(3,3); y = -2*eye(3,3); >> 10 = 'Sue'; y = 'Bob' >> x = 'Jane'; y = 'Bob'
Here is another simple function, traparea.m, with three input parameters and one output parameter. Since there is only one output parameter the foursquare brackets may exist omitted.
office area = traparea(a,b,h) % traparea(a,b,h) Computes the expanse of a trapezoid given % the dimensions a, b and h, where a and b % are the lengths of the parallel sides and % h is the distance between these sides % Compute the area, but suppress printing of the result area = 0.5*(a+b)*h;Note that at that place is a blank line between the annotate statements that describe the function and the single comment statement that describes the calculation of area. The comment statement commencement ``Compute the area...'' volition not exist printed if you lot type ``help traparea''. Also note that in that location is no ``return'' statement needed. (There is a MATLAB return argument, just it is not needed here.) The output variable,
area
, is defined in the first line of the file. The value assigned to area
is returned to the calling role or the control window. Finally, here is another unproblematic function, cart2plr.k, with two input parameters and two output parameters.
function [r,theta] = cart2plr(ten,y) % cart2plr Convert Cartesian coordinates to polar coordinates % % [r,theta] = cart2plr(x,y) computes r and theta with % % r = sqrt(x^2 + y^2); % theta = atan2(y,x); r = sqrt(ten^2 + y^two); theta = atan2(y,x);The annotate statements have empty lines, but these will be printed if y'all blazon ``help cart2plr''.
Brand sure MATLAB knows the path to your function
MATLAB cannot execute a office unless it knows where to discover its m-file. This requires that the function be in the internal MATLAB path. Refer to ``Setting the MATLAB Path'' for more data.Calling MATLAB Functions
- utilise or ignore render arguments (encounter also nargin/nargout)
- suppress output
Local Variables
Unless explicitly alleged to be global variables, all variables appearing in a MATLAB function are local to that role.Menstruation Control
MATLAB supports the bones menstruation control constructs found in most high level programming languages. The syntax is a hybrid of C and Fortran and I often create polyglot statements which lead to the joyless task of squashing trivial bugs.if constructs
MATLAB supports these variants of the ``if'' construct- if ... terminate
- if ... else ... stop
- if ... elseif ... else ... end
A uncomplicated warning
>> d = b^two - 4*a*c; >> if d<0 >> disp('warning: discriminant is negative, roots are imaginary'); >> endor a warning plus actress notification
>> d = b^two - 4*a*c; >> if d<0 >> disp('warning: discriminant is negative, roots are imaginary'); >> else >> disp('OK: roots are real, but may be repeated'); >> finishor, no secrets whatever
>> d = b^two - 4*a*c; >> if d<0 >> disp('warning: discriminant is negative, roots are imaginary'); >> elseif d==0 >> disp('discriminant is zip, roots are repeated'); >> else >> disp('OK: roots are existent and distinct'); >> endCareful inspection of the preceding statements reveals that
- no semicolon is needed to suppress output at the finish of lines containing if, else, elseif or endif
- elseif has no space between ``else'' and ``if''
- the finish argument is required
- the ``is equal to'' operator has 2 equals signs (see Logical Comparisons beneath)
The compact if
If you lot read tightly coded m-files (e.g., many of the built-in MATLAB functions) you lot will find a variant of the if ... end construct that is written on one line. Here'due south an example if 10<0, disp('imaginary'); endDetect the comma between the
x<0
and the disp(...)
. Manifestly the comma tells the MATLAB interpretter that the conditional examination has ended. To my noesis this is only place where a statement (OK, function of a statement) ends with a comma. It's just one of those quirks that true believers come to utilise without hesitation. Logical comparisons
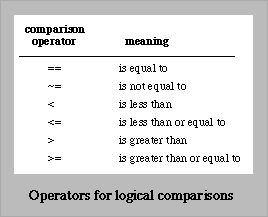
The preceding table lists the logical comparison operators used in MATLAB. Note that these operators can be used in assignment statements, as illustrated in this example
plenty = xnew-xold < delta if ~enough stepSize = stepSize/2; endThe start statement assigns the value of the logical comparison to the variable ``
enough
''. If the comparing on the correct hand side evaluates to be true, and so plenty
is given the value 1. If the comparison evaluates to be false, then enough
is given the value 0. In the subsequent if
statement, enough = 1
is true, and enough = 0
is false. Binary and unary logical operators
Binary operators take two arguments (operands). Unary operators take one argument. The &&
operator (read ``and'' operator) takes 2 logical expressions and returns ``true'' if both expressions are true, and ``false'' otherwise.
The ||
operator (read ``or'' operator) takes 2 logical expressions and returns ``true'' if either of the expressions are true, and ``false'' only if both expressions are simulated. The possible outcomes of &&
and ||
operations are summarized in the following truth tabular array.
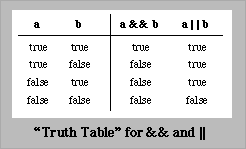
The ~
operator (read ``non'') takes merely ane logical expression and returns the opposite (negation) of that expression. Consider the post-obit code
a = two; b = iii; asmall = a < b; % asmall is ``truthful'' if ~asmall disp('a is greater than b') endSince
a
is less than b
the if ~asmall
block is not executed because ~asmall
is imitation. while constructs
The syntax of thewhile
construct is while expression do something endwhere ``expression'' is a logical expression. The ``practice something'' block of code is repeated until the expression in the while statement becomes false.
Hither is an example
i = 2; while i<eight i = i + 2 stopExecuting the preceding block of code results in the following output to the command window
i = 4 i = vi i = 8(The output has been adapted to take upwards less space. MATLAB likes to sprawl its printout over several lines.) Note that no
disp
or fprintf
argument was needed in the preceding block because the i = i + 2
statement did not end in a semicolon. for constructs
The for construct is used to create a loop, usually over a stock-still range of stepssum = 0; for i=i:length(10) while i<10 sum = sum + abs(x(i)); stop
[Preceding Section] [Chief Outline] [Department Outline] [Side by side Section]
etheridgepasto1975.blogspot.com
Source: https://web.cecs.pdx.edu/~gerry/MATLAB/programming/basics.html